Here's the situation:
I have a image (using a class already made) and i need to add to that image a film effect, that adds 2 black bars on each side of the image, and white squares on it, like this:
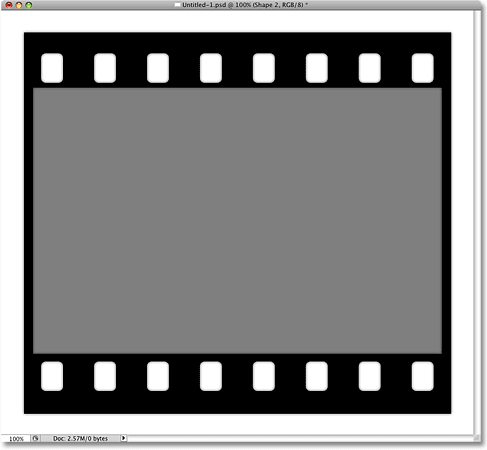
But in vertical, so i made a 2 for loops that will create the black bars (painting 1 black pixel from top to bottom) and its working 100%.
Now the white squares, what i did was something like this:
Code: Select all
for(int x = 7; x < black_bar_length - 7; x++)
{
for(int y = 10; y < img.getHeight(); y++)
{
if(y <= 35)
//Paint white dot at (x,y)
else if(y > 45 && y <= 70)
//Paint white dot at (x,y)
}
}
What you may have noticed is that i start at the y=10 (give a little margin) and then go till y = 35 so the square is 25x25.
What i want to do is some other (automatic) way to fill those white squares instead of write down EVERY single interval like i did till i reached 300px, but if the image is longer than that.. no white squares after 300px

Can you guys just point me in the right direction?
Thank you
